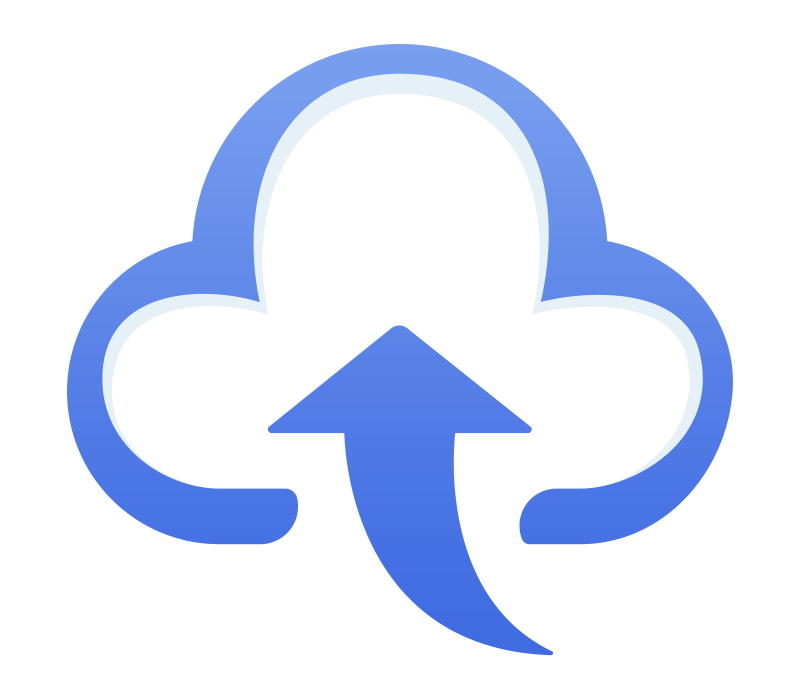
redgoose file uploader
플러그인은 업로드 컴포넌트의 필수요소는 아니지만 좀더 편하거나 강력한 기능을 덧붙이기 위하여 만들었습니다.
코어의 기능을 참조하여 프로그램의 기능을 추가할 수 있습니다.
Preview
업로드한 이미지 큐를 선택하면 왼쪽 영역에서 어떤 사진인지 확인할 수 있습니다.
var plug_preview = new RG_Uploader.core(document.getElementById('element_id'), {
queue : {
datas : 'data.json'
},
plugin : [
{ name : 'preview', obj: new RG_Uploader.plugins.Preview() }
]
});
drag & drop
큐 목록 공간으로 파일을 드래그 앤 드롭으로 집어넣으면 업로드를 할 수 있습니다.
다른 영역에 엘리먼트를 만들어서 거기로 파일을 넣을수도 있습니다.
set html element
<div class="rg-external-dropzone">external dropzone</div>
javascript code
var dnd_basic = new RG_Uploader.core(document.getElementById('element_id'), {
plugin : [
{ name : 'dnd', obj: new RG_Uploader.plugins.DragAndDrop() }
]
});
Size info
현재 올라온 파일들의 용량과 어느정도까지 업로드할 수 있는지 정보를 표시합니다.
var plug_sizeInfo = new RG_Uploader.core(document.getElementById('element_id'), {
queue : {
datas : 'data.json'
},
plugin : [
{ name : 'sizeInfo', obj: new RG_Uploader.plugins.SizeInfo() }
]
});
Change queue style
스위치 버튼을 눌러 queue 스타일을 바꿀 수 있습니다.
컴포넌트 헤더에 버튼이 위치해 있지만 css와 플러그인 js를 수정하여 고칠 수 있습니다.
File upload
아래 업로드 버튼을 눌러서 파일을 추가하거나 이 영역에 파일을 드래그하여 추가합니다.
var plug_changeQueueStyle = new RG_Uploader.core(document.getElementById('element_id'), {
queue : {
style : 'web',
datas : 'data.json'
},
plugin: [
{
name: 'changeQueueStyle',
obj: new RG_Uploader.plugins.ChangeQueueStyle({
endChangeItem: function(app) {
console.log('USER::endChangeItem', app.queue.items);
}
})
}
]
});
`ChangeQueueStyle` 플러그인은 Sortable 라이브러리를 사용하고 있습니다.
모듈형 환경에서 만든다면 Sortable 클래스 객체를 직접 불러올 수 있습니다.
// install package
npm install sortablejs --save
import Sortable from 'sortablejs';
// skip option
new RG_Uploader.plugins.ChangeQueueStyle({
class_sortable: Sortable
})
Make thumbnail image
첨부되어있는 사진 한장에서 이미지를 잘라내어 작은 이미지를 만들 수 있습니다.
기능을 적용한 이미지로 업로드할 수 있지만 다른 액션을 원하면 변경할 수 있습니다.
File upload
아래 업로드 버튼을 눌러서 파일을 추가하거나 이 영역에 파일을 드래그하여 추가합니다.
var plug_thumbnail = new RG_Uploader.core(document.getElementById('element_id'), {
queue : {
style : 'album',
height : 240,
datas : 'data.json',
buttons : [
{
name : 'make thumbnail image',
iconName : 'apps',
className : 'btn-make-thumbnail',
show : function(file) {
return (file.type.split('/')[0] == 'image');
},
action : function(app, file) {
if (!app.plugin.child.thumbnail) return false;
var plug = app.plugin.child.thumbnail;
plug.assignOption({ uploadScript : '' });
plug.open(file);
}
},
{
name : 'remove queue',
iconName : 'close',
className : 'btn-remove-queue',
action : function(app, file) {
app.queue.removeQueue(file.id, false, true);
}
}
]
},
plugin : [
{ name : 'thumbnail', obj: new RG_Uploader.plugins.Thumbnail() }
]
});
`Thumbnail` 플러그인은 Croppie 라이브러리를 사용하고 있습니다. 모듈형 환경에서 만든다면 Croppie 클래스 객체를 직접 불러올 수 있습니다.
// install package
npm install croppie --save
import croppie from 'croppie';
import 'croppie/croppie.css';
// skip option
new RG_Uploader.plugins.ChangeQueueStyle({
class_croppie: croppie.Croppie
})
change queue
업로드한 이미지 큐의 순서를 변경합니다.
var plug_changeQueue = new RG_Uploader.core(document.getElementById('element_id'), {
queue : {
height: null,
datas : 'data.json'
},
plugin : [
{
name: 'changeQueue',
obj: new RG_Uploader.plugins.ChangeQueue({
endChangeItem : function(app) {
console.log(app.queue.items);
}
})
}
]
});